How to use Error Provider Control in Windows Forms and C#
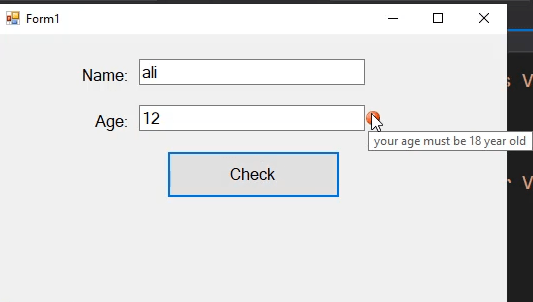
How to use Error Provider Control in Windows Forms and C#
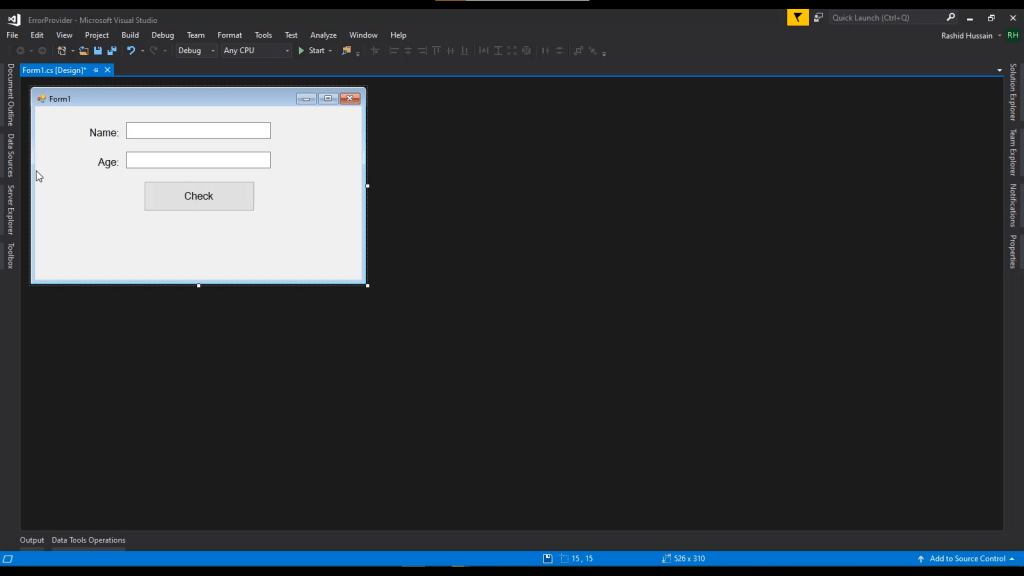
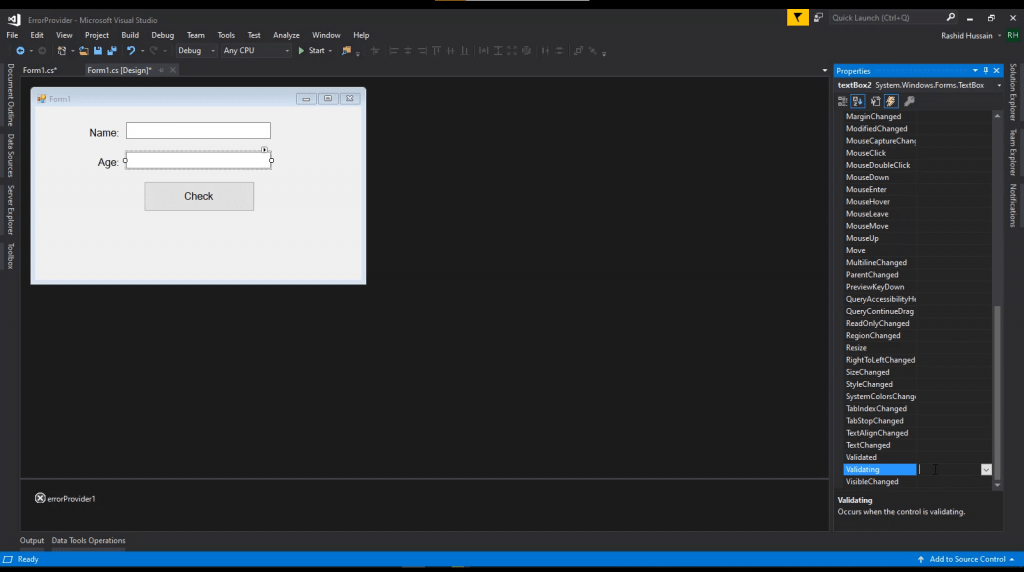
TextBox 1 Validating
private bool CheckName() { bool status = true; if (textBox1.Text=="") { errorProvider1.SetError(textBox1,"Please Enter Your Name"); status = false; } else { errorProvider1.SetError(textBox1,""); } return status; }
TextBox 2 Validating
private bool CheckAge() { bool status = true; if (textBox2.Text=="") { errorProvider1.SetError(textBox2,"Please Enter Your Age"); status = false; } else { errorProvider1.SetError(textBox2,""); try { int checkage = int.Parse(textBox2.Text); errorProvider1.SetError(textBox2, ""); if (checkage<18) { errorProvider1.SetError(textBox2, "your age must be 18 year old"); status = false; } else { errorProvider1.SetError(textBox2, ""); } } catch (Exception ex) { errorProvider1.SetError(textBox2, "Please enter your age as number"); status = false; } } return status; }
When the button is pressed, we will now add code to our sample to validate the entire form.
private void checkForm() { bool Name = CheckName(); bool age = CheckAge(); if (Name && age) { MessageBox.Show("Your Data Is Valid"); } else { MessageBox.Show("Please Enter Valid Data"); } }
Complete Code
using System; using System.Collections.Generic; using System.ComponentModel; using System.Data; using System.Drawing; using System.Linq; using System.Text; using System.Threading.Tasks; using System.Windows.Forms; namespace ErrorProvider { public partial class Form1 : Form { public Form1() { InitializeComponent(); } private void textBox1_Validating(object sender, CancelEventArgs e) { CheckName(); } private void textBox2_Validating(object sender, CancelEventArgs e) { CheckAge(); } private void Form1_Load(object sender, EventArgs e) { errorProvider1.ContainerControl = this; } private bool CheckName() { bool status = true; if (textBox1.Text=="") { errorProvider1.SetError(textBox1,"Please Enter Your Name"); status = false; } else { errorProvider1.SetError(textBox1,""); } return status; } private bool CheckAge() { bool status = true; if (textBox2.Text=="") { errorProvider1.SetError(textBox2,"Please Enter Your Age"); status = false; } else { errorProvider1.SetError(textBox2,""); try { int checkage = int.Parse(textBox2.Text); errorProvider1.SetError(textBox2, ""); if (checkage<18) { errorProvider1.SetError(textBox2, "your age must be 18 year old"); status = false; } else { errorProvider1.SetError(textBox2, ""); } } catch (Exception ex) { errorProvider1.SetError(textBox2, "Please enter your age as number"); status = false; } } return status; } private void checkForm() { bool Name = CheckName(); bool age = CheckAge(); if (Name && age) { MessageBox.Show("Your Data Is Valid"); } else { MessageBox.Show("Please Enter Valid Data"); } } private void button1_Click(object sender, EventArgs e) { checkForm(); } } }
Video tutorial
Thank you for visit. Please don’t forget to subscribe our official YouTube Channel RashiCode